Introduction
In this engaging tutorial, we will delve into how to store data using an I2C 32KB FRAM MB85RC256V with STM32 – Part 1, a powerful tool for the microcontroller community that can seamlessly store 32KB x 8 bits of data. Our journey begins with a straightforward example where we will guide you through the setup process, demonstrating how to write and read from this innovative memory device. Picture the possibilities: from capturing sensor data to storing essential information, the MB85RC256V opens up a realm of potential applications. Imagine using it to save images for display on an LCD module or managing application configuration parameters effortlessly. This device not only enhances your projects but also sparks your creativity, inviting you to explore its capabilities fully. We encourage you to share your experiences and seek support within the vibrant MicroControllersTech community, where collaboration and learning thrive. With an optimistic outlook, we invite you to experiment with the MB85RC256V memory, empowering you to broaden your technical skills and ignite your passion for hands-on innovation!
The upcoming tutorials promise to delve into a range of innovative and practical techniques for efficiently storing and retrieving blocks of data, utilizing structures and other C type-related methods. In addition, we will address an intriguing topic that has sparked discussion in various forums: the use of external storage while maintaining the ability of the compiler to generate addresses for global variables, with the actual data being stored externally. This critical area of exploration will be thoroughly covered in our future posts, empowering users to confidently navigate advanced data storage solutions in their projects. As we embark on this journey together, we encourage our community to engage, share insights, and collaboratively enhance our understanding of these essential concepts.
Materials List
- FTDI to USB
- NUCLEO-F439ZI
- MB85RC256V Memory IC 32KB Development Tools FRAM Breakout Board I2C Non-Volatile
- Breadboards Kit Include 2PCS 830 Point 2PCS 400 Point Solderless Breadboards
- Logic 8 – Saleae 8-Channel Logic Analyzer
FTDI Pinouts
FTDI to USB Pinout from right to left
- Pin 1 – GND
- Pin 2 – CTS
- Pin 3 – VCC
- Pin 4 – TX
- Pin 5 – RX
- Pin 6 – DTR
- USB Mini – Connect to PC via USB cable
Make sure the jumper is set for 5v or 3v which ever is being used to power the FTDI device. If we look below at the schematic, it is showing in this case as 5v.
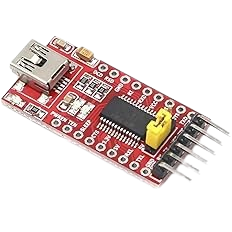
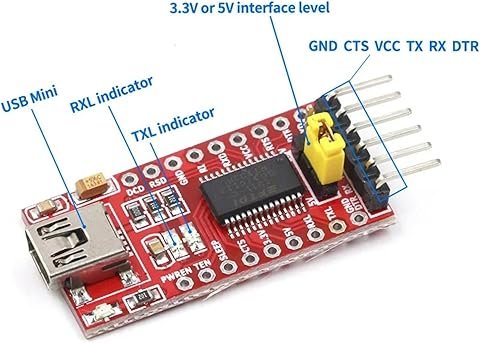
MB85RC256V Pinouts
MB85RC256V Memory IC 32KB FRAM
The AdaFruit breakout board is based upon the MB85RC256V chip.
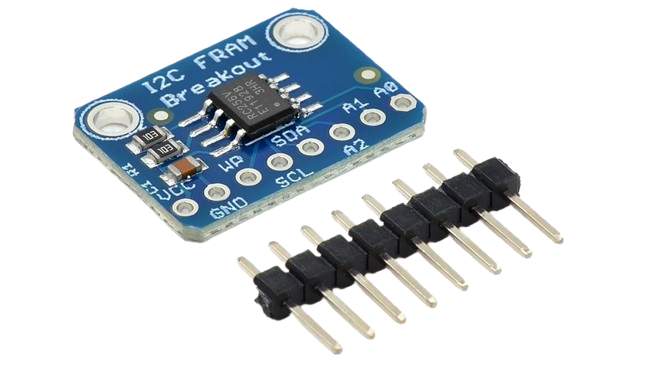
When working with I2C Devices it is import to ensure that the SCL and SDA lines are setup with physical pull-up resistors. Otherwise unexpected results and strange behavior could occur. Here is an article which pertains to I2C and Pull-Ups. Note, that on some STM32 MCU’s this can be done by setting the GPIO pins for the SCL/SDA lines to include pull-up resistors as parameters. Therefore, external resistors are not needed.
Schematic
Schematic to create the I2C 32KB FRAM MB85RC256V project
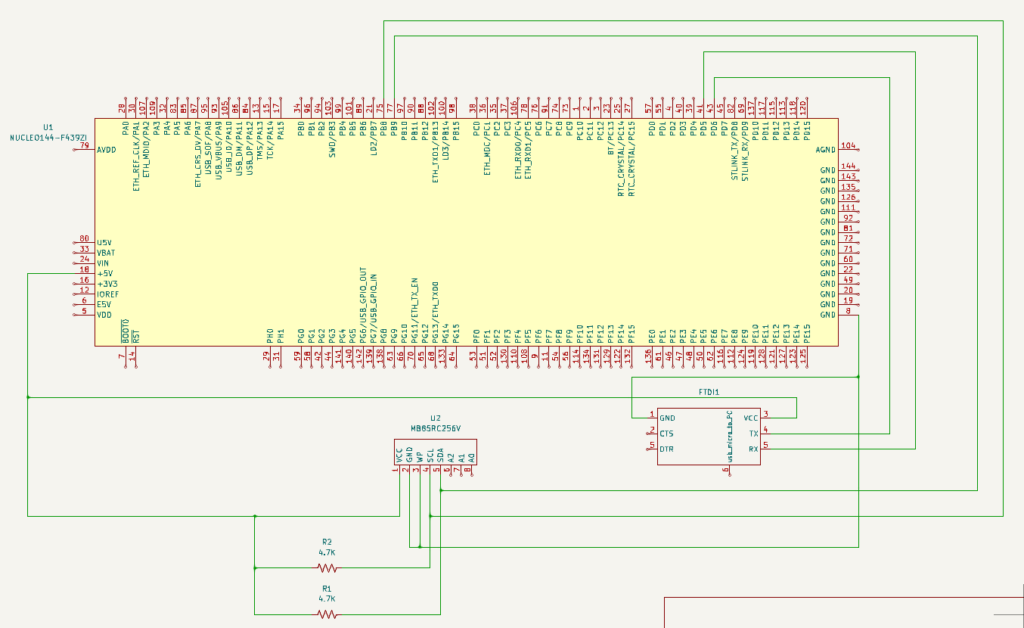
Schematic
Pinouts & Configurations
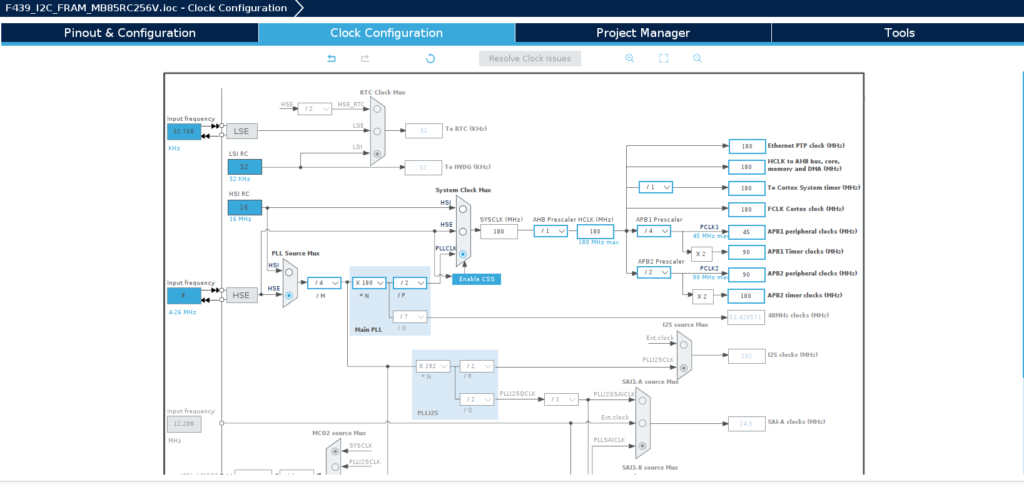
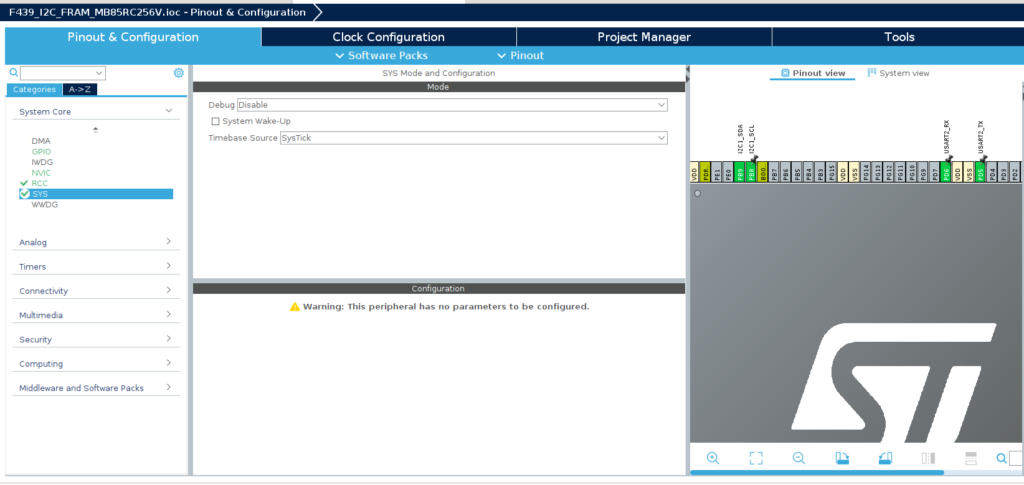
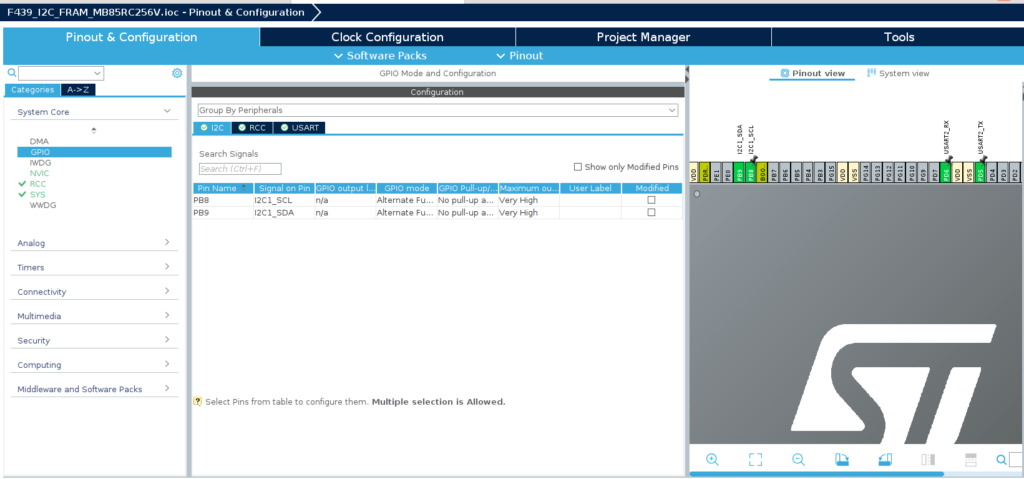
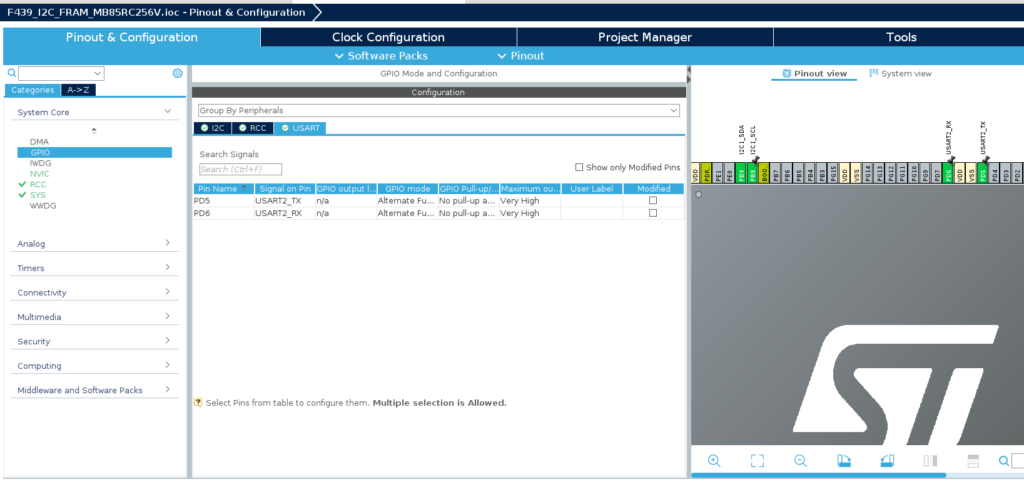
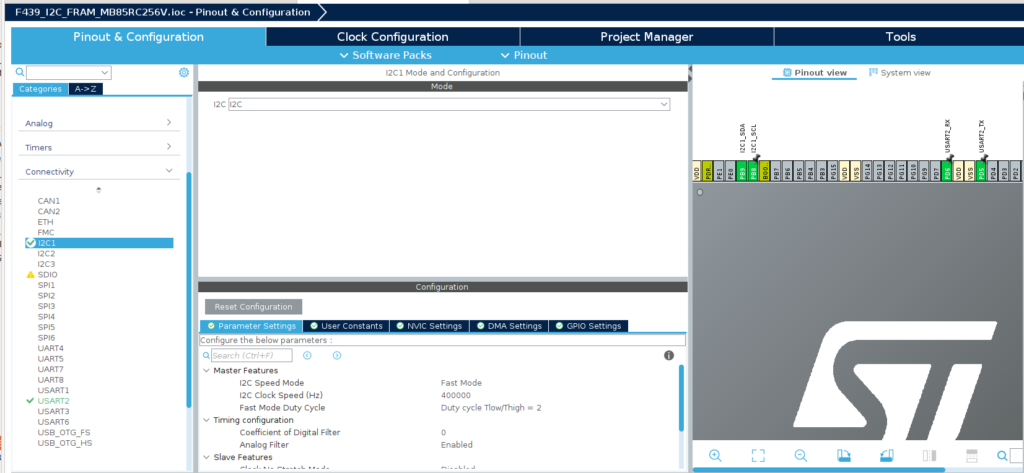
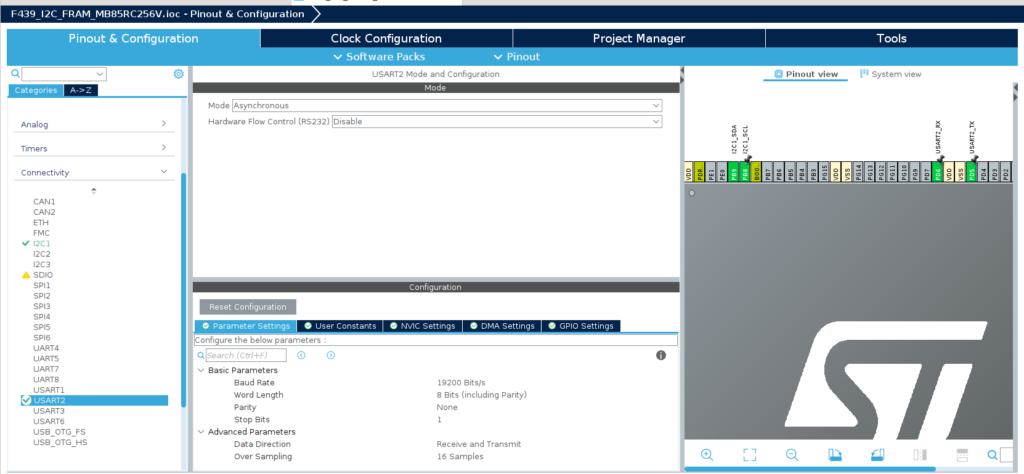
Code Listings for the I2C 32KB FRAM MB85RC256V project
main.c
/* USER CODE BEGIN Header */
/**
******************************************************************************
* @file : main.c
* @brief : Main program body
******************************************************************************
* @attention
*
* Copyright (c) 2024 STMicroelectronics.
* All rights reserved.
*
* This software is licensed under terms that can be found in the LICENSE file
* in the root directory of this software component.
* If no LICENSE file comes with this software, it is provided AS-IS.
*
******************************************************************************
*/
/* USER CODE END Header */
/* Includes ------------------------------------------------------------------*/
#include "main.h"
/* Private includes ----------------------------------------------------------*/
/* USER CODE BEGIN Includes */
#include <stdio.h>
#include "mb85rc256v.h"
/* USER CODE END Includes */
/* Private typedef -----------------------------------------------------------*/
/* USER CODE BEGIN PTD */
/* USER CODE END PTD */
/* Private define ------------------------------------------------------------*/
/* USER CODE BEGIN PD */
/* USER CODE END PD */
/* Private macro -------------------------------------------------------------*/
/* USER CODE BEGIN PM */
/* USER CODE END PM */
/* Private variables ---------------------------------------------------------*/
I2C_HandleTypeDef hi2c1;
UART_HandleTypeDef huart2;
/* USER CODE BEGIN PV */
/* USER CODE END PV */
/* Private function prototypes -----------------------------------------------*/
void SystemClock_Config(void);
static void MX_GPIO_Init(void);
static void MX_I2C1_Init(void);
static void MX_USART2_UART_Init(void);
/* USER CODE BEGIN PFP */
/* USER CODE END PFP */
/* Private user code ---------------------------------------------------------*/
/* USER CODE BEGIN 0 */
#ifdef REDIRECT_PRINTF
#define PUTCHAR_PROTOTYPE int __io_putchar(int ch)
#endif
#ifdef REDIRECT_PRINTF
/**
* @brief Retargets the C library printf function to the USART.
* @param None
* @retval None
*/
PUTCHAR_PROTOTYPE
{
/* Place your implementation of fputc here */
/* e.g. write a character to the USART2 and Loop until the end of transmission */
HAL_UART_Transmit(&huart2, (uint8_t *)&ch, 1, 0xFFFF);
return ch;
}
#endif
void simpleTest() {
HAL_StatusTypeDef halStatus = HAL_OK;
int len = 0;
char rcvBuf[128];
char buffer[128];
printf("Starting simpleTest\r\n");
memset(rcvBuf, 0x00, sizeof(rcvBuf));
memset(buffer, 0x00, sizeof(buffer));
halStatus = HAL_I2C_IsDeviceReady(&hi2c1, (uint16_t) MB85rc_ADDRESS, 5, 10);
if (halStatus == HAL_OK) {
sprintf(buffer, "Now is the time for all good men to come to the aid of their country!!!!\r\n");
len = strlen(buffer) + 1;
halStatus = MB85rc_WriteByte(0x00, (uint8_t *) &buffer, len);
if (halStatus != HAL_OK) {
Error_Handler();
}
} else {
Error_Handler();
}
if (halStatus == HAL_OK) {
halStatus = MB85rc_ReadByte(0x00, (uint8_t *) &rcvBuf, len);
if (halStatus != HAL_OK) {
Error_Handler();
}
}
printf(rcvBuf);
printf("Ending simpleTest\r\n");
}
/* USER CODE END 0 */
/**
* @brief The application entry point.
* @retval int
*/
int main(void)
{
/* USER CODE BEGIN 1 */
/* USER CODE END 1 */
/* MCU Configuration--------------------------------------------------------*/
/* Reset of all peripherals, Initializes the Flash interface and the Systick. */
HAL_Init();
/* USER CODE BEGIN Init */
/* USER CODE END Init */
/* Configure the system clock */
SystemClock_Config();
/* USER CODE BEGIN SysInit */
/* USER CODE END SysInit */
/* Initialize all configured peripherals */
MX_GPIO_Init();
MX_I2C1_Init();
MX_USART2_UART_Init();
/* USER CODE BEGIN 2 */
#ifdef REDIRECT_PRINTF
printf("\x1b[2J\x1b[H"); // Clear the dumb terminal screen
#endif
MB85rc_Init(&hi2c1);
/* USER CODE END 2 */
/* Infinite loop */
/* USER CODE BEGIN WHILE */
while (1)
{
simpleTest();
printf("End of Tests\r\n");
fflush(stdout);
HAL_Delay(2000);
/* USER CODE END WHILE */
/* USER CODE BEGIN 3 */
}
/* USER CODE END 3 */
}
/**
* @brief System Clock Configuration
* @retval None
*/
void SystemClock_Config(void)
{
RCC_OscInitTypeDef RCC_OscInitStruct = {0};
RCC_ClkInitTypeDef RCC_ClkInitStruct = {0};
/** Configure the main internal regulator output voltage
*/
__HAL_RCC_PWR_CLK_ENABLE();
__HAL_PWR_VOLTAGESCALING_CONFIG(PWR_REGULATOR_VOLTAGE_SCALE1);
/** Initializes the RCC Oscillators according to the specified parameters
* in the RCC_OscInitTypeDef structure.
*/
RCC_OscInitStruct.OscillatorType = RCC_OSCILLATORTYPE_HSE;
RCC_OscInitStruct.HSEState = RCC_HSE_ON;
RCC_OscInitStruct.PLL.PLLState = RCC_PLL_ON;
RCC_OscInitStruct.PLL.PLLSource = RCC_PLLSOURCE_HSE;
RCC_OscInitStruct.PLL.PLLM = 4;
RCC_OscInitStruct.PLL.PLLN = 180;
RCC_OscInitStruct.PLL.PLLP = RCC_PLLP_DIV2;
RCC_OscInitStruct.PLL.PLLQ = 7;
if (HAL_RCC_OscConfig(&RCC_OscInitStruct) != HAL_OK)
{
Error_Handler();
}
/** Activate the Over-Drive mode
*/
if (HAL_PWREx_EnableOverDrive() != HAL_OK)
{
Error_Handler();
}
/** Initializes the CPU, AHB and APB buses clocks
*/
RCC_ClkInitStruct.ClockType = RCC_CLOCKTYPE_HCLK|RCC_CLOCKTYPE_SYSCLK
|RCC_CLOCKTYPE_PCLK1|RCC_CLOCKTYPE_PCLK2;
RCC_ClkInitStruct.SYSCLKSource = RCC_SYSCLKSOURCE_PLLCLK;
RCC_ClkInitStruct.AHBCLKDivider = RCC_SYSCLK_DIV1;
RCC_ClkInitStruct.APB1CLKDivider = RCC_HCLK_DIV4;
RCC_ClkInitStruct.APB2CLKDivider = RCC_HCLK_DIV2;
if (HAL_RCC_ClockConfig(&RCC_ClkInitStruct, FLASH_LATENCY_5) != HAL_OK)
{
Error_Handler();
}
}
/**
* @brief I2C1 Initialization Function
* @param None
* @retval None
*/
static void MX_I2C1_Init(void)
{
/* USER CODE BEGIN I2C1_Init 0 */
/* USER CODE END I2C1_Init 0 */
/* USER CODE BEGIN I2C1_Init 1 */
/* USER CODE END I2C1_Init 1 */
hi2c1.Instance = I2C1;
hi2c1.Init.ClockSpeed = 400000;
hi2c1.Init.DutyCycle = I2C_DUTYCYCLE_2;
hi2c1.Init.OwnAddress1 = 0;
hi2c1.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT;
hi2c1.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE;
hi2c1.Init.OwnAddress2 = 0;
hi2c1.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE;
hi2c1.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE;
if (HAL_I2C_Init(&hi2c1) != HAL_OK)
{
Error_Handler();
}
/** Configure Analogue filter
*/
if (HAL_I2CEx_ConfigAnalogFilter(&hi2c1, I2C_ANALOGFILTER_ENABLE) != HAL_OK)
{
Error_Handler();
}
/** Configure Digital filter
*/
if (HAL_I2CEx_ConfigDigitalFilter(&hi2c1, 0) != HAL_OK)
{
Error_Handler();
}
/* USER CODE BEGIN I2C1_Init 2 */
/* USER CODE END I2C1_Init 2 */
}
/**
* @brief USART2 Initialization Function
* @param None
* @retval None
*/
static void MX_USART2_UART_Init(void)
{
/* USER CODE BEGIN USART2_Init 0 */
/* USER CODE END USART2_Init 0 */
/* USER CODE BEGIN USART2_Init 1 */
/* USER CODE END USART2_Init 1 */
huart2.Instance = USART2;
huart2.Init.BaudRate = 19200;
huart2.Init.WordLength = UART_WORDLENGTH_8B;
huart2.Init.StopBits = UART_STOPBITS_1;
huart2.Init.Parity = UART_PARITY_NONE;
huart2.Init.Mode = UART_MODE_TX_RX;
huart2.Init.HwFlowCtl = UART_HWCONTROL_NONE;
huart2.Init.OverSampling = UART_OVERSAMPLING_16;
if (HAL_UART_Init(&huart2) != HAL_OK)
{
Error_Handler();
}
/* USER CODE BEGIN USART2_Init 2 */
/* USER CODE END USART2_Init 2 */
}
/**
* @brief GPIO Initialization Function
* @param None
* @retval None
*/
static void MX_GPIO_Init(void)
{
/* USER CODE BEGIN MX_GPIO_Init_1 */
/* USER CODE END MX_GPIO_Init_1 */
/* GPIO Ports Clock Enable */
__HAL_RCC_GPIOC_CLK_ENABLE();
__HAL_RCC_GPIOH_CLK_ENABLE();
__HAL_RCC_GPIOD_CLK_ENABLE();
__HAL_RCC_GPIOB_CLK_ENABLE();
/* USER CODE BEGIN MX_GPIO_Init_2 */
/* USER CODE END MX_GPIO_Init_2 */
}
/* USER CODE BEGIN 4 */
/**
* @brief This function is executed in case of error occurrence.
* @param file: The file name as string.
* @param line: The line in file as a number.
* @retval None
*/
void _Error_Handler(const char *file, int line)
{
/* USER CODE BEGIN Error_Handler_Debug */
__disable_irq();
#ifdef REDIRECT_PRINTF
char buf[80];
sprintf(buf, "Trapped in Error_Handler(). Called from: %s, line: %d\r\n", file, line);
printf(buf);
#endif
/* User can add his own implementation to report the HAL error return state */
while(1)
{
}
}
/* USER CODE END 4 */
#ifdef USE_FULL_ASSERT
/**
* @brief Reports the name of the source file and the source line number
* where the assert_param error has occurred.
* @param file: pointer to the source file name
* @param line: assert_param error line source number
* @retval None
*/
void assert_failed(uint8_t *file, uint32_t line)
{
/* USER CODE BEGIN 6 */
/* User can add his own implementation to report the file name and line number,
ex: printf("Wrong parameters value: file %s on line %d\r\n", file, line) */
/* USER CODE END 6 */
}
#endif /* USE_FULL_ASSERT */
main.h
/* USER CODE BEGIN Header */
/**
******************************************************************************
* @file : main.h
* @brief : Header for main.c file.
* This file contains the common defines of the application.
******************************************************************************
* @attention
*
* Copyright (c) 2024 STMicroelectronics.
* All rights reserved.
*
* This software is licensed under terms that can be found in the LICENSE file
* in the root directory of this software component.
* If no LICENSE file comes with this software, it is provided AS-IS.
*
******************************************************************************
*/
/* USER CODE END Header */
/* Define to prevent recursive inclusion -------------------------------------*/
#ifndef __MAIN_H
#define __MAIN_H
#ifdef __cplusplus
extern "C" {
#endif
/* Includes ------------------------------------------------------------------*/
#include "stm32f4xx_hal.h"
/* Private includes ----------------------------------------------------------*/
/* USER CODE BEGIN Includes */
/* USER CODE END Includes */
/* Exported types ------------------------------------------------------------*/
/* USER CODE BEGIN ET */
/* USER CODE END ET */
/* Exported constants --------------------------------------------------------*/
/* USER CODE BEGIN EC */
/* USER CODE END EC */
/* Exported macro ------------------------------------------------------------*/
/* USER CODE BEGIN EM */
/* USER CODE END EM */
/* Exported functions prototypes ---------------------------------------------*/
void Error_Handler(void);
/* USER CODE BEGIN EFP */
/* USER CODE END EFP */
/* Private defines -----------------------------------------------------------*/
/* USER CODE BEGIN Private defines */
void _Error_Handler(const char *, int);
#define Error_Handler() _Error_Handler((const char *)__FILE__, __LINE__)
#define REDIRECT_PRINTF
/* USER CODE END Private defines */
#ifdef __cplusplus
}
#endif
#endif /* __MAIN_H */
Device driver for I2C 32KB FRAM MB85RC256V project
mb85rc256v.h
/*
* mb85rc256v.h
*
* Created on: Jul 29, 2024
* Author: jpgroulx
*/
#include <string.h>
#include "main.h"
#ifndef MB85RC256V_H_
#define MB85RC256V_H_
/* MB85rc Register ----------------------------------------------------------*/
#define MB85rc_ADDRESS (0x50<<1) // mb85rc256v default address
#define MB85rc_EEPROM_SIZE 0x8000 // EEPROM Size 32768 bytes
/* MB85rc External Function -------------------------------------------------*/
void MB85rc_Init(I2C_HandleTypeDef *hi2c);
HAL_StatusTypeDef MB85rc_Bus_Write(uint16_t DevAddr, uint16_t MemAddr, uint8_t *pData, uint16_t Len);
HAL_StatusTypeDef MB85rc_Bus_Read(uint16_t DevAddr, uint16_t MemAddr, uint8_t *pData, uint16_t Len);
HAL_StatusTypeDef MB85rc_ReadByte(uint16_t MemAddr, uint8_t *pData, uint16_t Len);
HAL_StatusTypeDef MB85rc_WriteByte(uint16_t MemAddr, uint8_t *value, uint16_t Len);
#ifdef __cplusplus
}
#endif
#endif /* MB85RC256V_H_ */
mb85rc256v.c
/*
* mb85rc256v.c
*
* Created on: Jul 29, 2024
* Author: johng
*/
#include "mb85rc256v.h"
#include "stdio.h"
I2C_HandleTypeDef *i2c;
/**
* @brief Initialize local I2C handle
*/
void MB85rc_Init(I2C_HandleTypeDef *hi2c) {
i2c = hi2c;
}
/**
* @brief I2C Bus Write 16bit
* @retval HAL_StatusTypeDef
* @param DevAddr Target device address
* @param memAddr Internal memory address
* @param pData Pointer to data buffer
* @param Len Amount of data to be Write
*/
HAL_StatusTypeDef MB85rc_Bus_Write(uint16_t DevAddr, uint16_t memAddr, uint8_t *pData, uint16_t Len)
{
HAL_StatusTypeDef halStatus = HAL_OK;
halStatus = HAL_I2C_Mem_Write(i2c, DevAddr, memAddr, I2C_MEMADD_SIZE_16BIT, pData, Len, HAL_MAX_DELAY);
return halStatus;
}
/**
* @brief I2C Bus Read 16bits
* @retval HAL_StatusTypeDef
* @param DevAddr Target device address
* @param memAddr Internal memory address
* @param pData Pointer to data buffer
* @param Len Amount of data to be read
*/
HAL_StatusTypeDef MB85rc_Bus_Read(uint16_t DevAddr, uint16_t memAddr, uint8_t *pData, uint16_t Len)
{
HAL_StatusTypeDef halStatus = HAL_OK;
halStatus = HAL_I2C_Mem_Read(i2c, DevAddr, memAddr, I2C_MEMADD_SIZE_16BIT, pData, Len, HAL_MAX_DELAY);
return halStatus;
}
/**
* @brief Sequential read from selected memory address with number of byte
* @retval HAL_StatusTypeDef
* @param memAddr Memory address to start read from
* @param pData Pointer to data
* @param Len Number of byte to read
*/
uint8_t MB85rc_ReadByte(uint16_t memAddr, uint8_t *pData, uint16_t Len)
{
HAL_StatusTypeDef halStatus = HAL_OK;
halStatus = MB85rc_Bus_Read(MB85rc_ADDRESS, memAddr, pData, Len);
return halStatus;
}
/**
* @brief Write data into EEPROM
* @retval HAL_StatusTypeDef
* @param memAddr Memory address to start write from
* @param pData Pointer to data
* @param Len Number of byte to write
*/
uint8_t MB85rc_WriteByte(uint16_t memAddr, uint8_t *value, uint16_t Len)
{
HAL_StatusTypeDef halStatus = HAL_OK;
halStatus = MB85rc_Bus_Write(MB85rc_ADDRESS, memAddr, value, Len);
return halStatus;
}
USART Output
Terminal emulator output window
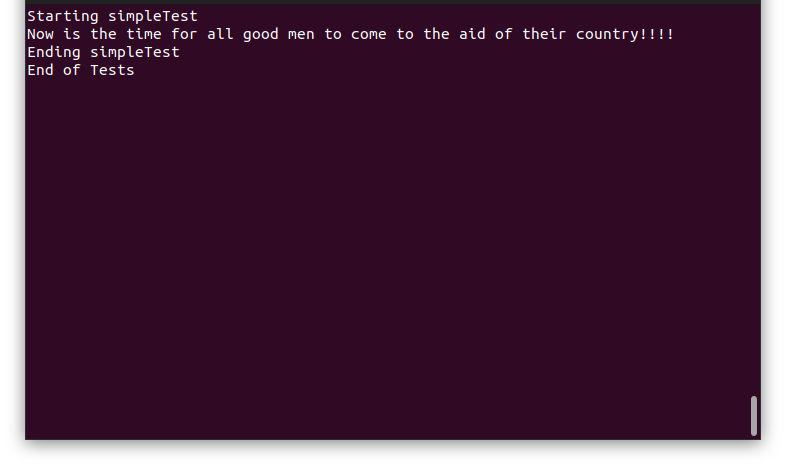
Logic Analyzer Output
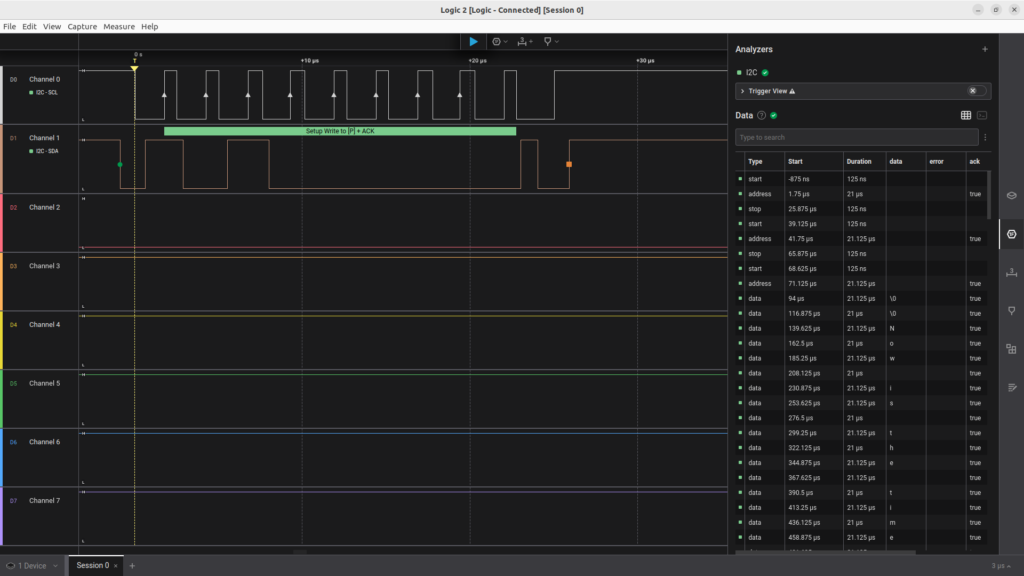
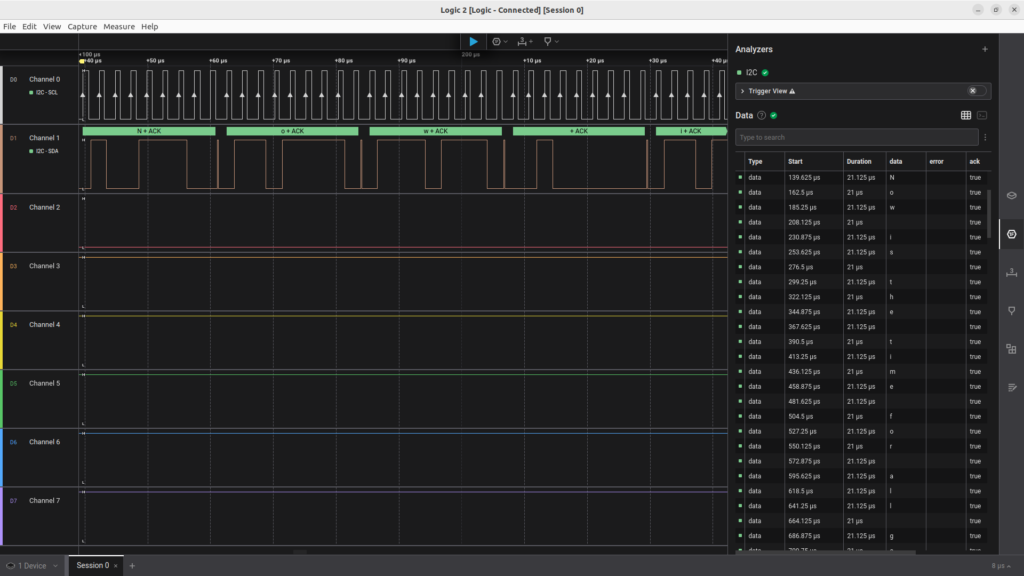
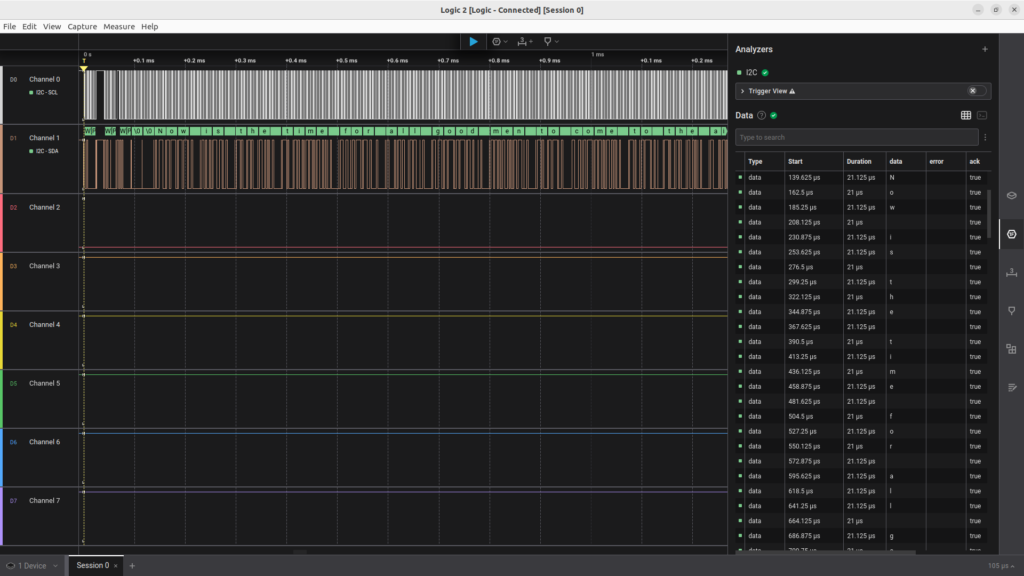